· 2 min read
React-three-fiber with Drei and react-spring
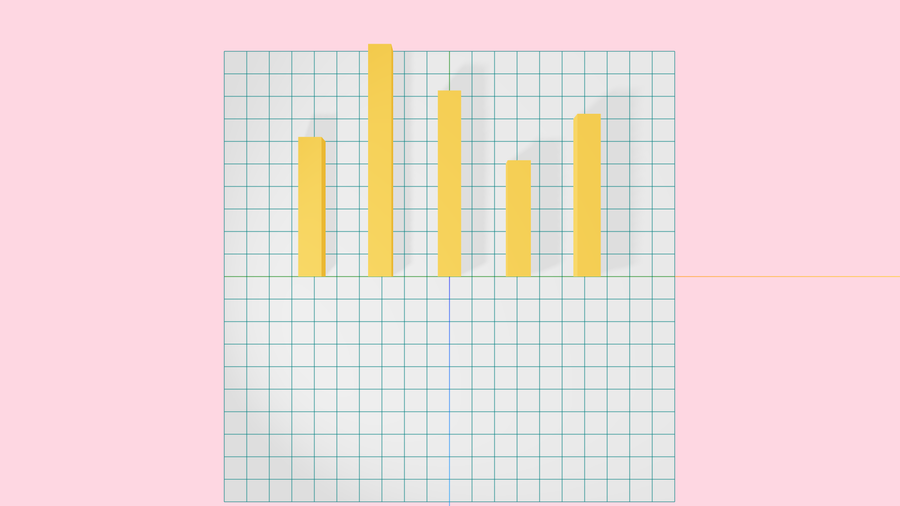
Now that the actors and lights are in place, let’s add some action(animation) to the scene. The react-spring library is a fantastic library for creating animations in React. It is based on the spring physics model and allows us to create some really cool animations with minimal code.
As I’ll be working on data visualizations in the future, let’s see how we can create a simple animated bar chart using all these awesome libraries.
The juicy part of the code is in the R3fChart component. Let’s take a look at it.
const items = [300, 500, 400, 250, 350];
const [springs, api] = useSprings(items.length, (i) => ({
from: { position: [(i - 2) * 3, 0.5, 0], scale: [1, 1, 1] },
}));
const showChart = () => {
api.start((i) => ({
to: {
position: [(i - 2) * 3, 0.5, -items[i] / 100],
scale: [1, 1, items[i] / 50],
},
}));
};
const hideChart = () => {
api.start((i) => ({
to: { position: [(i - 2) * 3, 0.5, 0], scale: [1, 1, 1] },
}));
};
The items array contains the values for the bars. The useSprings hook creates an array of spring objects. The first parameter is the number of springs to create, and the second parameter is a function that returns the initial values for each spring. The initial values are the position and scale of the bar. The position is calculated by multiplying the index of the bar with 3 and subtracting 2. This is done to center the bars around the origin. The scale is set to 1 for all bars.
The two eventHandlers showChart and hideChart are used to start the animation. The showChart function scales the bars, however, as the scale function grows the bar on bot sides, we need to simultaneously adjust the postion, to make the bars look like they are growing from the initiation postion. I wish there was a better method to do this, but this seems to be the simplest way as of now.
The hideChart function simply resets the position and scale of the bar to the initial values.
And then we create the bars using the springs as below
{
springs.map((animation, i) => (
<AnimatedBox key={i} castShadow {...animation}>
<meshStandardMaterial color="orange" />
</AnimatedBox>
));
}
The spring effects (slow start, then fast, then slow again) are thanks to the react-spring library and can be adjusted as per the requirements.